40 python plot with labels
Matplotlib X-axis Label - Python Guides Use the xlabel () method in matplotlib to add a label to the plot's x-axis. Let's have a look at an example: # Import Library import matplotlib.pyplot as plt # Define Data x = [0, 1, 2, 3, 4] y = [2, 4, 6, 8, 12] # Plotting plt.plot (x, y) # Add x-axis label plt.xlabel ('X-axis Label') # Visualize plt.show () Python Plot Multiple Lines Using Matplotlib - Python Guides Plot the data (multiple lines) and adding the features you want in the plot (title, color pallete, thickness, labels, annotation, etc…). Show the plot (graph/chart). You can also save the plot. Let's plot a simple graph containing two lines in python. So, open up your IPython shell or Jupiter notebook, and follow the code below:
How to Add Labels in a Plot using Python? - GeeksforGeeks Creating Labels for a Plot By using pyplot () function of library we can add xlabel () and ylabel () to set x and y labels. Example: Let's add Label in the above Plot Python import matplotlib import matplotlib.pyplot as plt import numpy as np x = np.array ( [0, 1, 2, 3]) y = np.array ( [3, 8, 1, 10]) plt.plot (x, y)
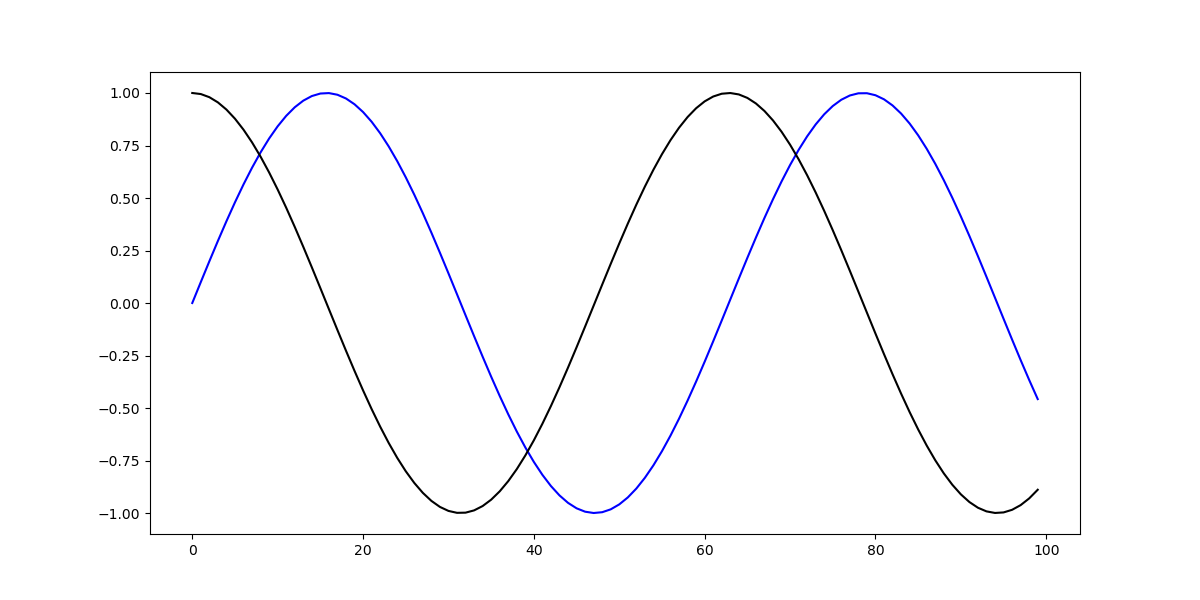
Python plot with labels
Adding value labels on a Matplotlib Bar Chart - GeeksforGeeks For Plotting the bar chart with value labels we are using mainly two methods provided by Matplotlib Library. For making the Bar Chart Syntax: plt.bar (x, height, color) For adding text on the Bar Chart Syntax: plt.text (x, y, s, ha, Bbox) We are showing some parameters which are used in this article: Steps Needed: Import the library. matplotlib.pyplot.plot — Matplotlib 3.6.0 documentation Plotting multiple sets of data. There are various ways to plot multiple sets of data. The most straight forward way is just to call plot multiple times. Example: >>> plot(x1, y1, 'bo') >>> plot(x2, y2, 'go') Copy to clipboard. If x and/or y are 2D arrays a separate data set will be drawn for every column. Python Matplotlib Implement a Scatter Plot with Labels: A Completed ... First, we will check the length of coord and labels are the same or not by python assert statement. To understand assert statement, you can read this tutorial. Then, we will use plt.scatter (x, y) to draw these scatter points. Finally, we will use plt.annotate () function to display labels of these scatter points.
Python plot with labels. matplotlib.pyplot.legend — Matplotlib 3.6.0 documentation Use this together with labels, if you need full control on what is shown in the legend and the automatic mechanism described above is not sufficient. The length of handles and labels should be the same in this case. If they are not, they are truncated to the smaller length. labels list of str, optional. A list of labels to show next to the artists. Line chart with labels at end of each line - The Python Graph Gallery A custom lineplot with annotations at the end of each line to explore the evolution of the Big Mac Index with Python and Matplotlib.From the data preparation to the final layout customization, this blogpost will guide you through all the steps to produce a beautiful lineplot with labeled groups. python matplotlib for loop plot with function and labels import numpy as np import matplotlib.pyplot as plt x = np.linspace (0, 10, 100) y = [ [5*x], [1*x], [2*x]] labels = ['foo', 'bar', 'baz'] for y_arr, label in zip (y, labels): plt.plot (x, y_arr, label=label) plt.legend () plt.show () python matplotlib Share asked Oct 30, 2017 at 12:40 KaZyKa 316 2 10 2 Python Scatter Plot - Python Geeks Python Scatter Plot. Scatter plot in Python is one type of a graph plotted by dots in it. The dots in the plot are the data values. To represent a scatter plot, we will use the matplotlib library. To build a scatter plot, we require two sets of data where one set of arrays represents the x axis and the other set of arrays represents the y axis ...
Matplotlib Bar Chart Labels - Python Guides The syntax to plot bar chart and define labels on the x-axis are as follow: # Plot bar chart matplotlib.pyplot.bar (x, height) # Define x-axis labels matplotlib.pyplot.xlabels () The parameters defined above are outlined as below: x: specifies x-coordinates of the bar. height: specifies y-coordinates of the bar. Matplotlib Labels and Title - W3Schools With Pyplot, you can use the xlabel () and ylabel () functions to set a label for the x- and y-axis. Example Add labels to the x- and y-axis: import numpy as np import matplotlib.pyplot as plt x = np.array ( [80, 85, 90, 95, 100, 105, 110, 115, 120, 125]) y = np.array ( [240, 250, 260, 270, 280, 290, 300, 310, 320, 330]) plt.plot (x, y) How to add text labels to a scatterplot in Python? - Data Plot Plus Python Add text labels to Data points in Scatterplot The addition of the labels to each or all data points happens in this line: [plt.text (x=row ['avg_income'], y=row ['happyScore'], s=row ['country']) for k,row in df.iterrows () if 'Europe' in row.region] We are using Python's list comprehensions. Iterating through all rows of the original DataFrame. Hover text and formatting in Python - Plotly Hovermode x or y¶. If layout.hovermode='x' (or 'y'), a single hover label appears per trace, for points at the same x (or y) value as the cursor.If multiple points in a given trace exist at the same coordinate, only one will get a hover label. In the line plot below we have forced markers to appear, to make it clearer what can be hovered over, and we have disabled the built-in Plotly Express ...
python - Plot graph with vertical labels on the x-axis Matplotlib ... Yes you can, with xticks (). # Your labels list labels = [chr (x) for x in range (65, 91)] # > ["A", "B", "C" ..., "Z"] as an example import numpy as np data = np.random.rand (26, 1) # random data to illustrate import matplotlib.pyplot as plt plt.xticks (ticks=range (len (data)), labels=labels, rotation=90) plt.plot (data) # Your data plt.show () Legends, Titles, and Labels with Matplotlib - Python Programming To start: import matplotlib.pyplot as plt x = [1,2,3] y = [5,7,4] x2 = [1,2,3] y2 = [10,14,12] This way, we have two lines that we can plot. Next: plt.plot(x, y, label='First Line') plt.plot(x2, y2, label='Second Line') Here, we plot as we've seen already, only this time we add another parameter "label." 7 ways to label a cluster plot in Python — Nikki Marinsek Seaborn makes it incredibly easy to generate a nice looking labeled scatter plot. This style works well if your data points are labeled, but don't really form clusters, or if your labels are long. #plot data with seaborn facet = sns.lmplot(data=data, x='x', y='y', hue='label', fit_reg=False, legend=True, legend_out=True) STYLE 2: COLOR-CODED LEGEND How to Add Text Labels to Scatterplot in Python (Matplotlib/Seaborn ... However when it comes to scatter plots, these python libraries do not have any straight forward option to display labels of data points. This feature is available in other data visualization tools like Tableau and Power BI, with just a few clicks or hovering the pointer over the datapoints. ... Basic scatter plot Label Specific Items.
Graph Plotting in Python | Set 1 - GeeksforGeeks Give a name to x-axis and y-axis using .xlabel () and .ylabel () functions. Give a title to your plot using .title () function. Finally, to view your plot, we use .show () function. Plotting two or more lines on same plot Python import matplotlib.pyplot as plt # line 1 points x1 = [1,2,3] y1 = [2,4,1] plt.plot (x1, y1, label = "line 1")
Matplotlib.pyplot.legend() in Python - GeeksforGeeks Matplotlib is one of the most popular Python packages used for data visualization. It is a cross-platform library for making 2D plots from data in arrays. Pyplot is a collection of command style functions that make matplotlib work like MATLAB. Each pyplot function makes some change to a figure: e.g., creates a figure, creates a plotting area in a figure, plots some lines in a plotting area ...
Python Matplotlib Implement a Scatter Plot with Labels: A Completed ... First, we will check the length of coord and labels are the same or not by python assert statement. To understand assert statement, you can read this tutorial. Then, we will use plt.scatter (x, y) to draw these scatter points. Finally, we will use plt.annotate () function to display labels of these scatter points.
matplotlib.pyplot.plot — Matplotlib 3.6.0 documentation Plotting multiple sets of data. There are various ways to plot multiple sets of data. The most straight forward way is just to call plot multiple times. Example: >>> plot(x1, y1, 'bo') >>> plot(x2, y2, 'go') Copy to clipboard. If x and/or y are 2D arrays a separate data set will be drawn for every column.
Adding value labels on a Matplotlib Bar Chart - GeeksforGeeks For Plotting the bar chart with value labels we are using mainly two methods provided by Matplotlib Library. For making the Bar Chart Syntax: plt.bar (x, height, color) For adding text on the Bar Chart Syntax: plt.text (x, y, s, ha, Bbox) We are showing some parameters which are used in this article: Steps Needed: Import the library.
Post a Comment for "40 python plot with labels"